C# 8 提供了許多新語法,很多新語法可以讓我們的程式碼閱讀性提高,但前提是我們要先了解有那些新功能
這裡我先挑出一個我最近才用到的好用功能,陣列索引
索引
早期在操作陣列取值時,使用 Linq
應該算是標準解,但假設只是要取陣列最後的幾個項目,使用 Linq
似乎又太囉嗦
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| using System; using System.Linq;
namespace csharp { class Program { static void Main(string[] args) { var urls = "https://blog.kevinyang.net/images/4550568.jpg".Split('/');
var imagesFile = urls.Last(); var folderName = urls.SkipLast(1).LastOrDefault(); Console.WriteLine("Image FileName: {0}", imagesFile); Console.WriteLine("Folder Name: {0}", folderName); } } }
|
執行結果
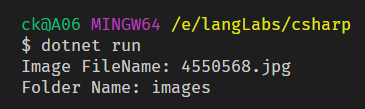
如果是使用 C# 8.0 的新語法,就可以這樣子寫
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| using System;
namespace csharp { class Program { static void Main(string[] args) { var urls = "https://blog.kevinyang.net/images/4550568.jpg".Split('/');
var imagesFile = urls[^1]; var folderName = urls[^2]; Console.WriteLine("Image FileName: {0}", imagesFile); Console.WriteLine("Folder Name: {0}", folderName); } } }
|
說明
新語法,當使用 ^
,索引計算起點就會從最後面開始起算,圖解如下
1 2 3 4 5 6 7 8 9 10 11 12 13
| var words = new string[] { "The", "quick", "brown", "fox", "jumped", "over", "the", "lazy", "dog" };
|
範圍
既然知道如何使用索引表示,接下來是另外一個新功能,範圍
。這一個功能在某些語言已經存在一段時間了
語法表示
Array[StartIndex … EndIndex(不包含 End Index 的值)]
範例 (延續上面的 words
陣列)
1 2
| var lazyDogs = words[^2..^0]; var firstThreeWords = words[0..3]
|
當不指定開始或結束索引時,就會是為最頭至最尾
1 2 3
| var allwords = words[..]; var firstThreeWords = words[..3]; var skipFirstThree = words[3..];
|
當然也可以先決定 Range
型別後,在放到陣列中
1 2 3 4 5 6 7 8
| var range0 = 1..2; var range1 = new Range(1, 2); var range2 = Range.StartAt(3); var range3 = Range.EndAt(3); Console.WriteLine(String.Join(',', words[range0])); Console.WriteLine(String.Join(',', words[range1])); Console.WriteLine(String.Join(',', words[range2])); Console.WriteLine(String.Join(',', words[range3]));
|
以下型別都有支援索引和範圍的新功能
參考資料